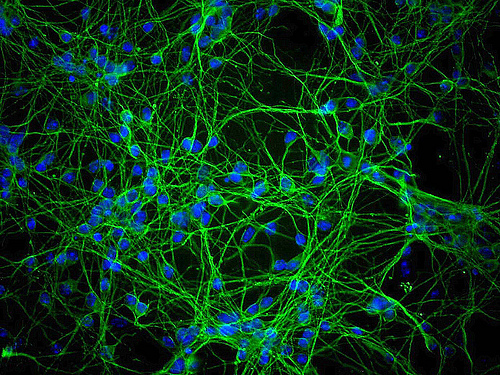
I have recently released backbone-signal, which is a reactive programming (Wikipedia) library with a practical & javascripty model api.
// backbone-signal extends Backbone.Model
var app = new Backbone.Model();
var userSignal = app.signal("user");
userSignal.getTruthy(app, function(app, user) {
console.info("Hello " + user.name);
});
console.info("Let's see some friends");
userSignal.set({
name: "Jane"
});
userSignal.getTruthy(app, function(app, user) {
console.info("Nice to see you");
});
userSignal.set({
name: "Joe"
});
userSignal.unset();
The console ouput is:
Let's see some friends
Hello Jane
Nice to see you
Hello Joe
Nice to see you
We are calling getTruthy
on the userSignal
two times, one for "Hello " + user.name
and one for "Nice to see you"
. The callback is invoked when the value is Truthy. So when userSignal.unset
is called, the callbacks are not invoked.
What is nice about having a dedicated signal object is that you can bind to it even when it's value is undefined, thereby avoiding order dependencies and simplifying your logic.
backbone-signal also utilizes Backbone's listenTo
and listenToOnce
methods, which make it easy to clean up by calling stopListening
on the listener.
backbone-signal is being used in Rundavoo and has been fun to use, especially with Node.js & Browserify. It's been a pleasure using a lightweight unframework to freely structure the dataflow logic of the site.
The api includes:
Loading/Unloading
load
- Invokes the loader when the value is not definedforceLoad
- Invokes the loader (regardless if the value is defined)reload
- Unsets the value then invokes the loaderunload
- Invokes the unloadersetLoader
- Sets the Loader callbackunsetLoader
- Unsets the Loader callbacksetUnloader
- Sets the Unloader callbackunsetUnloader
- Unsets the Unloader callback
Setters
set
- Sets the value with the argumentunset
- Unets the valuevalue
- Returns the value
Getters/Listeners
get
- Invoke the callback immediately and on any additional changes to the valuelisten
- Listen to any additional changes to the value (does not invoke the callback immediately)getOnce
- Invoke the callback immediately one timelistenOnce
- Listen to any additional changes to the value one timegetTruthy
- Invoke the callback immediately and on any additional changes to the value if the value is truthylistenTruthy
- Listen to any additional changes to the value if the value is truthygetTruthyOnce
- Invoke the callback immediately or on any additional changes to the value if the value is truthy one time onlylistenTruthyOnce
- Listen to any additional changes to the value if the value is truthy one time only- getFalsy- Invoke the callback immediately and on any additional changes to the value if the value is falsy
listenFalsy
- Listen to any additional changes to the value if the value is falsygetFalsyOnce
- Invoke the callback immediately or on any additional changes to the value if the value is falsy one time onlylistenFalsyOnce
- Listen to any additional changes to the value if the value is falsy one time only- getDefined- Invoke the callback immediately and on any additional changes to the value if the value is defined
listenDefined
- Listen to any additional changes to the value if the value is definedgetDefinedOnce
- Invoke the callback immediately or on any additional changes to the value if the value is defined one time onlylistenDefinedOnce
- Listen to any additional changes to the value if the value is defined one time onlyunbind
- Unbinds the given object from the callbackloading
isLoading